If you've come within 30 feet of the internet this last month, you'll have come across
this list of best practices at least a dozen times. Everybody seems to be writing about it and linking to it and
building little tools that tell you you're not doing it right.
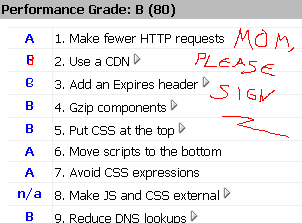
Most of the stuff on that list is low hanging fruit. You can spend 5 minutes in IIS, flipping compression on and telling all your /images/ directories not to expire content until we're all driving flying cars, and suddenly you'll find your site loading a lot faster.
That's cool and all, but what if you also followed their advice and stuck a bunch of your static content out on Amazon S3? I guess you just fire up
S3Fox and start playing with the metadata on all those… whoa, hang on… hey, you can't change that stuff once it's written. Crap. You've gotta upload all those files again. And you can't use that cool Firefox tool to do it anymore, because it has no way to set an "Expires" header when you upload a file. Crap. Crap. Crap.
Well if you're running C# and ASP.NET, you're in luck. Because I just went through that pain for
a few of my sites, and now I'm going to let you mooch off my code.
First step: download the right library from Amazon
In this case, you're going to need the
Amazon S3 REST Library for C#. No, not the SOAP library, because evidently
that one is crap. Either drop the source straight into your project or build it elsewhere and link it in.
Last step: swipe this code
This zip contains everything you'll need. Just airlift it into your project and you'll be good to go. Now, since this is an article about programming, I'm legally obligated to provide at least one code sample for you to gloss over. So here is the meat of what we're doing:
public void PushToAmazonS3ViaREST(string bucket, string relativePath, HttpServerUtility server)
{
relativePath = relativePath.TrimStart('/');
string fullPath = _basePath + relativePath.Replace(@"/", @"\");
AWSAuthConnection s3 = new AWSAuthConnection(_publicKey, _secretKey);
string sContentType = "image/jpeg";
SortedList sList = new SortedList();
sList.Add("Content-Type", sContentType);
// Set access control list to "publicly readable"
sList.Add("x-amz-acl", "public-read");
// Set to expire in ten years
sList.Add("Expires", GetHttpDateString(DateTime.Now.AddYears(10)));
S3Object obj = new S3Object(FileContentsAsString(fullPath), sList);
s3.PutObjectAsStream(bucket, relativePath, fullPath, obj.Metadata);
}
There's only two lines you need to care about if you're using S3 to host web content, and they're both commented. One sets the file to be readable by the public, and the other tells it not to expire until after you've left the company. Sorted.
I've included a cheesy .aspx page that you can use to push your files by hand. Hopefully you can figure out how to change which directories it's putting in the list, and how to add your own. It's actually pretty ugly code, but hey, it's just an admin tool that you'll only run a few times in your life.
Be Warned though: I've stripped out the security that keeps people from the outside world (and GoogleBot) from hitting this page and bogging your server. If there's any chance that this might escape to the live site, be sure to lock it down so that you can't see it unless you're logged in as an admin!
Anyway, I hope you find some use out of that code. I certainly wasn't planning to publish it, so please refrain from mentioning the 47-odd things in it that you
should never do in production!
Enjoy!
paint chat software
by
Jason Kester
Discuss on hacker news